Unity游戏暂停功能概述
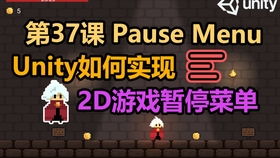
在Unity游戏开发中,暂停功能是一个常见且重要的功能。它允许玩家在游戏中暂时停止游戏进程,进行一些操作,如查看游戏菜单、保存游戏进度等。本文将详细介绍Unity中实现游戏暂停功能的方法和技巧。
使用Time.timeScale实现暂停
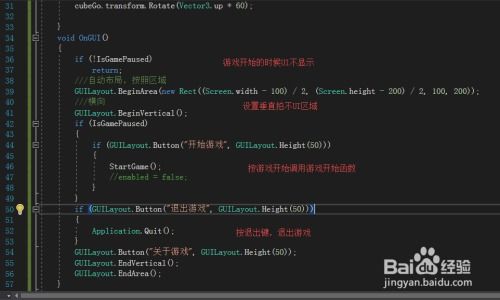
Unity中,暂停游戏最常用的方法是使用Time.timeScale属性。Time.timeScale是一个浮点数,用于控制游戏的时间流逝速度。当Time.timeScale的值为0时,游戏将暂停;当Time.timeScale的值为1时,游戏恢复正常速度。
以下是一个简单的暂停和恢复游戏状态的示例代码:
```csharp
using UnityEngine;
public class GamePause : MonoBehaviour
public GameObject pauseMenu;
void update()
{
if (Input.GetKeyDown(KeyCode.Escape))
{
if (Time.timeScale == 1)
{
PauseGame();
}
else
{
ResumeGame();
}
}
}
void PauseGame()
{
pauseMenu.SetActive(true);
Time.timeScale = 0;
}
void ResumeGame()
{
pauseMenu.SetActive(false);
Time.timeScale = 1;
}
处理Time.timeScale对游戏的影响
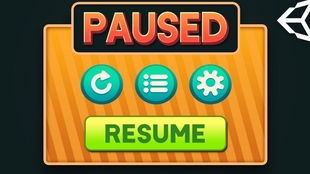
当Time.timeScale为0时,游戏中的物理事件和依赖时间的函数都会暂停。这意味着,如果游戏中有物体正在运动,它们会立即停止。但是,update和LateUpdate函数仍然会执行,这意味着游戏仍然在渲染,只是看起来没有变化。
在暂停时,禁用或启用游戏中的物体,而不是直接停止它们的运动。
使用协程(Coroutine)来处理暂停和恢复游戏状态,确保在暂停期间不会执行不必要的操作。
在暂停时,保存游戏进度,以便玩家可以在恢复游戏时继续。
实现暂停菜单
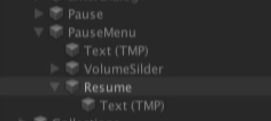
暂停菜单是暂停功能的重要组成部分。以下是一个简单的暂停菜单实现方法:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class PauseMenu : MonoBehaviour
public GameObject pauseMenu;
public Button resumeButton;
public Button quitButton;
void Start()
{
resumeButton.onClick.AddListener(ResumeGame);
quitButton.onClick.AddListener(QuitGame);
}
void ResumeGame()
{
pauseMenu.SetActive(false);
Time.timeScale = 1;
}
void QuitGame()
{
Application.Quit();
}
处理Time.deltaTime在暂停时的值
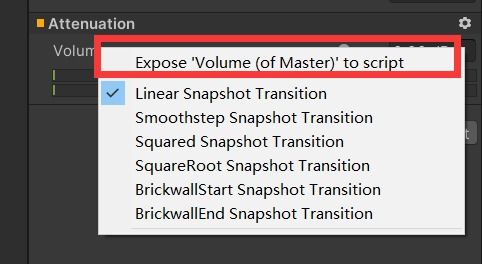
当Time.timeScale为0时,Time.deltaTime的值也为0。这意味着,在暂停期间,依赖于Time.deltaTime的函数(如动画、平滑移动等)将不会执行。为了解决这个问题,可以在暂停时使用一个固定的deltaTime值。
```csharp
using UnityEngine;
public class SmoothMovement : MonoBehaviour
public float speed = 5f;
private float fixedDeltaTime = 0.0167f; // 60 frames per second
void update()
{
if (Time.timeScale == 1)
{
transform.Translate(Vector3.forward speed Time.deltaTime);
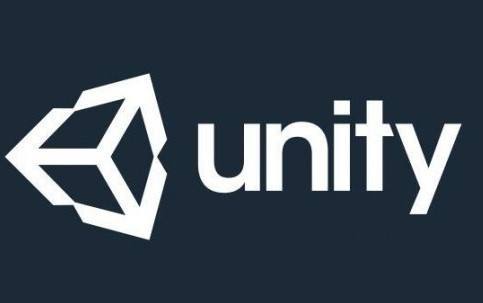
}
else
{
transform.Translate(Vector3.forward speed fixedDeltaTime);
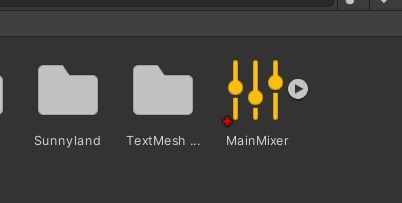
}
}
Unity游戏暂停功能是游戏开发中不可或缺的一部分。通过使用Time.timeScale和相关的技巧,可以轻松实现游戏暂停和恢复功能。本文介绍了使用Time.timeScale实现暂停、处理Time.timeScale对游戏的影响、实现暂停菜单以及处理Time.deltaTime在暂停时的值等技巧,希望对Unity游戏开发者有所帮助。